How to Add CAPTCHA in Codeigniter
In this example, we will show you how to add CAPTCHA in Codeigniter.
- CAPTCHA is randomly generated string in an image file & stored in SESSION variable. CodeIgniter provides a CAPTCHA helper to generate random code.
Captcha Code Form –
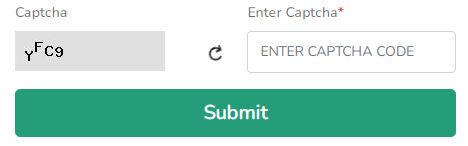
Controller (Captchaform.php) –
- The create_captcha() function generate random CAPTCHA Code as image & stored a SESSION variable.
- The captcha_refresh() function generate random CAPTCHA code after click on the refresh button as show on the above figure.
- After click on the submit button, check_captcha() function checks the CAPTCHA code is correct or not.
defined('BASEPATH') OR exit('No direct script access allowed');
class Captchaform extends CI_Controller {
public function __construct() {
parent::__construct();
// Load captcha helper
$this->load->helper('captcha');
// Load session library
$this->load->library('session');
$this->load->helper('form');
// form validation
$this->load->library('form_validation');
}
public function index() {
// Code for Captcha form is submitted
if($this->input->post('submit')){
$this->form_validation->set_rules('captcha', 'Captcha', 'required|callback_check_captcha');
}
$captchaconfig = array(
'img_path' => 'captcha_images/',
'img_url' => base_url() . 'captcha_images/',
'img_width' => '150',
'img_height' => 40,
'font_size' => 16,
'word_length' => 4,
'expiration' => 7200,
'colors' => array(
'background' => array(224, 224, 224),
'border' => array(224, 224, 224),
'text' => array(0, 0, 0),
'grid' => array(224, 224, 224)
),'pool' => '123456789ABCDEFGHIJKLMNPQRSTUVWXYZ',
);
$data['captcha'] = create_captcha($captchaconfig);
$_SESSION['captchaWord'] = $data['captcha']['word'];
}
public function captcha_refresh() {
// Captcha configuration
$random_number = substr(number_format(time() * rand(), 0, '', ''), 0, 4);
$captchaconfig = array(
'img_path' => 'captcha_images/',
'img_url' => base_url() . 'captcha_images/',
'img_width' => '150',
'img_height' => 40,
'font_size' => 16,
'word_length' => 4,
'expiration' => 7200,
'colors' => array(
'background' => array(224, 224, 224),
'border' => array(224, 224, 224),
'text' => array(0, 0, 0),
'grid' => array(224, 224, 224)
),
'pool' => '123456789ABCDEFGHIJKLMNPQRSTUVWXYZ',
);
$data['captcha'] = create_captcha($captchaconfig);
$this->session->unset_userdata('captchaWord');
$this->session->set_userdata('captchaWord', $data['captcha']['word']);
// Display captcha image
echo $data['captcha']['image'];
}
// define function for check captcha code
public function check_captcha($str) {
$word = $this->session->userdata('captchaWord');
if (strcmp(strtoupper($str), strtoupper($word)) == 0) {
return true;
} else {
$this->form_validation->set_message('check_captcha', 'Please enter correct words!');
return false;
}
}
public function validateCaptcha() {
$captcha_insert = $this->input->post('captcha');
$contain_sess_captcha = $this->session->userdata('captchaWord');
if ($captcha_insert == $_SESSION['captchaWord'] ) {
$this->session->set_userdata('captchaVerified', 'Yes');
echo 1;
} else {
echo 0;
}
}
}
View (index.php) –
- This code “$captcha[‘image’]” loads the CAPTCHA code.
<!-- add jquery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<div>
<h3>Add Captcha in Codeigniter</h3>
</div>
<form action="#" method="post" autocomplete="off">
<div>
<label>Captcha</label>
<span class="captcha"><?php echo $captcha['image']; ?> </span>
</div>
<div class="cp_refresh">
<a href="javascript:void(0);" class="captcha-refresh" title="Refresh Captcha"><span class="fas fa-redo"></span></a>
</div>
<div>
<label>Enter Captcha</label>
<input id ="captchid" type="text" style="text-transform:uppercase" name="captcha" placeholder="Enter Captcha Code">
<?php echo form_error('captcha', '<div class="alert-danger"><p>', '</p></div>'); ?>
</div>
<div>
<input type="submit" name="submit" value="Submit">
</div>
</form>